Installation
Simple Method
Download the latest release here- Extract the CSS and JavaScript files from the dist folder to your project folder.
- WebUI has no dependencies, so you don't need to include jQuery.
- In your web page, add a link to the CSS in the head section and a link to the JavaScript file at the bottom of the body section, as the following example:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title></title> <link rel="stylesheet" href="lib/webui/dist/css/webui-components-standard.css"> </head> <body> <script src="js/webui-components.min.js"></script> </body> </html>
Using a CDN
If you prefer not to download the full package and just want to try it out, then this can be done by linking to the required files on a CDN as follows.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title></title> <link rel="stylesheet" href="https://unpkg.com/asyncdesign-webui@latest/dist/css/webui-standard.min.css"> </head> <body> <script src="https://unpkg.com/asyncdesign-webui@latest/dist/js/webui-components.min.js"></script> </body> </html>
NPM
This is the recommended way to install WebUI if you are building websites using popular frameworks such as Angular, React, or Vue.
npm install asyncdesign-webui
Yarn
Alternatively, you can use Yarn to install the WebUI packages.
yarn add asyncdesign-webui
NuGet
If you are using Visual Studio before VS2019 to develop websites, then this is the preferred way to install WebUI.
Install-Package asyncdesign-webui
LibMan
If you are using Visual Studio 2019 or later, then the built-in "LibMan" utility is the best way to manage client-side libraries.
- That's it. You are ready to start developing with WebUI.
- Be sure to look at the static DEMO pages at the root of the download, containing many examples that demonstrate a good selection of components.
What's Included?
NPM
The NPM package includes the WebUI source files and the minified and development distribution files.
Yarn
Yarn installs the same package as with NPM, but may be better suited to certain projects or environments.
NuGet
The NuGet package includes only the minified and development distribution files.
GitHub Download
The GitHub download includes all WebUI files for the framework, docs website, demo pages, and build files.
Components Available JavaScript required
The following components are included in WebUI.
- Alerts
- Animation
- Carousels
- FocusTrap
- Menus
- Modals
- Navbar
- NavButton
- NavIndicator
- Positioning
- Radial
- Scrollspy
- Shapes
- Tabs
- ToastContainer
- ToggleContainer
- Tooltips
- Upload
- Validation
- Zoom
Building WebUI
Build Steps
The NPM package manager requires node to be installed first. You will need Node 18.x or later. The latest version can be downloaded here. Node Download
NPM is installed by the Node installer. This will install the latest NPM globally. For more information see NPM installation docs here. NPM Installation
Now you are ready to set up the WebUI project. Start by downloading and unpacking the latest WebUI version or fork the WebUI GitHub repository to your chosen project folder. You can download the latest version of WebUI here. WebUI Latest
Open a command prompt and navigate to the project folder where you unpacked the WebUI project files.
Type the following and wait for dependencies to be installed.
npm install
This will install all packages listed in the package.json file, including WebUI.
To build the project for development, type the following:
npm run dev
To build the project for production, type the following:
npm run build
For more information on editing source files and building the project, see the WebUI Build section.
Useage
CSS and JavaScript files
WebUI comes with a range of pre-built CSS and JavaScript files. See below for the combinations that can be used and what is included in each.
CSS | JS | Details |
---|---|---|
webui-basic.min.css | webui.min.js (Optional) | Includes basic styles only. Does not include standard styles, advanced styles or WebUI components. |
webui-standard.min.css | webui.min.js (Optional) | Includes basic and standard styles. Does not include advanced styles or WebUI components. |
webui-all.min.css | webui.min.js (Optional) | Includes basic, standard, and advanced styles. Does not include WebUI components. |
webui-components-basic.min.css | webui-components.min.js (Required) | Includes basic styles only, and WebUI components. |
webui-components-standard.min.css | webui-components.min.js (Required) | Includes basic and standard styles, and WebUI components. |
webui-components-all.min.css | webui-components.min.js (Required) | Includes basic, standard, and advanced styles, and WebUI components. |
JavaScript DOM Ready Event
All WebUI JavaScript should be enclosed in the DOM ready event callback, as shown in the following example.
Use the optional flag set to true to ensure the page is fully loaded and the document.readyState is "complete" before running scripts. By default, the webui.ready event is called when the document DOMContentLoaded event is fired.
DOM Ready EventNOTE: The webui.ready event does not need to be used if you are using a framework lifecycle mounted / render event.
webui.ready( () => { // Call WebUI functions here... });
webui.ready( () => { // Call WebUI functions here... }, true);
Angular
- From the command line, navigate to your Angular CLI project folder.
- Type
npm install asyncdesign-webui
and wait for the install to finish. - In tsconfig.json add
"allowJs": true
to the "compilerOptions" object. - In .angular-cli.json add
"../node_modules/asyncdesign-webui/dist/css/webui-standard.min.css"
to the styles array. - In .angular-cli.json add
"../node_modules/asyncdesign-webui/dist/js/webui.min.js"
to the scripts array. - In typings.d.ts add
declare var webui: any
- In your app.components.ts add
import * as webui from '../../node_modules/asyncdesign-webui/dist/js/webui.min.js';
at the top of your file.
React
- From the command line, navigate to your React project folder.
- Type
npm install asyncdesign-webui
and wait for the install to finish. - In your React component js file such as App.js add
import webui from '../node_modules/asyncdesign-webui/dist/js/webui.min.js'
- Then add
import '../node_modules/asyncdesign-webui/dist/css/webui-standard.min.css'
- Don't forget to use
className=""
instead ofclass=""
on your html elements. - If you need to use WebUI javascript functions, this should be done in the
componentDidMount()
lifecycle method.
Vue
- From the command line, navigate to your Vue CLI project folder.
- Type
npm install asyncdesign-webui
and wait for the install to finish. - In webpack.base.conf.js under resolve: alias: add
'webui': resolve('node_modules/asyncdesign-webui/dist/js/webui.min.js')
- In main.js add
import webui from 'webui';
- In main.js add
import '../node_modules/asyncdesign-webui/dist/css/webui-standard.min.css';
- Then finally, add
Vue.use(webui)
directly below your imports.
WebUI Layouts
WebUI uses a 24 column flexbox grid system to create layouts. Each row can contain up to 24 columns, and each column can contain any other content.
In this example, the logo and heading use columns that apply regardless of breakpoints. Using the class col-fixed means that this column only takes the width needed for its content, while the col class causes that column to take the remaining row width. The navigation and content columns use proportional sizes (6 and 18), so the combined width is 24 columns. These columns are only applied at breakpoint 3 and over, hence the addition of -bp-3-over. When the viewport width is below breakpoint 3, both columns will expand to 100% width and stack vertically. You can also apply padding to all columns by adding a col-padding-* class to a container element.
<div class="container color-light background-info-dark col-padding-sm"> <div class="row"> <div class="col-fixed border-xs border-info"> Logo </div> <div class="col text-center border-xs border-info"> Heading </div> </div> <div class="row"> <div class="col-6-bp-3-over border-xs border-info"> Navigation </div> <div class="col-18-bp-3-over border-xs border-info"> Content </div> </div> </div>
For more information on breakpoints, see the Media Breakpoints section.
A responsive gutter can be added to the layout using one of the responsive-gutter-* classes to prevent the content from becoming too wide and keeping it centered.
For more information on the grid system and responsive gutters, see the WebUI Layout section.
Responsive GuttersResponsive gutters should not be applied directly to columns, but may be applied as a layout wrapper, to a row, or to elements within columns.
Flexbox
WebUI includes a 24 size flex item system to create flexbox layouts - this is a more direct way to use flexbox and is an alternative to the WebUI layout grid system. Flex items are sized using flex-basis and therfore operate either vertically or horizontally, depending on the flex direction.
As with WebUI layouts, flexbox classes also have breakpoint equivalents. More information on breakpoints can be found in the Media Breakpoints section.
Pellentesque habitant morbi tristique senectus et netus et malesuada fames ac turpis egestas. Vestibulum tortor quam, feugiat vitae, ultricies eget, tempor sit amet, ante.
- Alerts
- Carousel
- Menus
<div class="container color-light background-info-dark"> <div class="flex-row"> <div class="flex-col border-xs border-info text-center pad-sm"> <b>Heading</b> </div> </div> <div class="flex-row"> <div class="flex-size-18 flex-respond-bp-2-under border-xs border-info pad-md"> <b>Main</b> <p>Pellentesque habitant morbi tristique senectus et netus et malesuada fames ac turpis egestas. Vestibulum tortor quam, feugiat vitae, ultricies eget, tempor sit amet, ante.</p> </div> <div class="flex-size-6 order-first-bp-2-under flex-respond-bp-2-under border-xs border-info pad-md"> <b>Navigation</b> <p> <ul class="list gap-vertical-sm"> <li>Alerts</li> <li>Carousel</li> <li>Menus</li> </ul> </p> </div> </div> </div>
Using the flex-respond-bp-2-under class causes the flex-basis to become 100% when the viewport width is at breakpoint 2 or less, so that the column will take the full width of the row. The visual order of the "Navigation" section is set to appear before the "Main" section also when the viewport width is at breakpoint 2 or less.
It's very easy to create flexbox layouts with WebUI by using flexbox utility classes such as (flex-size-*, flex-auto, flex-initial, etc.).
Here are some examples.
<div class="flex text-sm"> <div class="control-label-sm accent-2 rounded-left-sm pad-left-sm pad-right-sm"> Label </div> <input type="text" class="input-sm flex-auto" /> <button type="button" class="button-sm button-accent-2 rounded-right-sm">✗</button> </div>
<div class="flex width-lg-xl height-xl light rounded-md shadow-sm"> <div class="flex-col flex-grow"> <header class="info-dark height-lg rounded-top-sm pad-sm">Header</header> <div class="flex-auto pad-sm">Content.</div> <footer class="rounded-bottom-sm text-xs pad-sm"> Footer </footer> </div> </div>
For more information on using flexbox see the Flexbox section.
Adding Content
The most basic content of any website is the text, and WebUI makes it easy to produce good formatting. Firstly, it's a good idea to add the text class to the body or section element of your page - this will ensure good readability by increasing the text line height.
Heading 1
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Vestibulum enim urna, sagittis et sollicitudin non, sollicitudin a neque. Nulla vitae.<div class="text text-md"> <h1>Heading 1</h1> Lorem ipsum dolor sit amet, consectetur adipiscing elit. Vestibulum enim urna, sagittis et sollicitudin non, sollicitudin a neque. Nulla vitae. </div>
Text SizesNOTE: The text sizing classes, e.g. text-lg, depend on the inherited size. So if a parent element has a text sizing class applied, then text-lg could appear smaller or larger for child elements.
For more information on formatting text see the Typography section.
Similarly, when adding an image the image class is very useful, as it will ensure the image scales with the size of it's containing parent element while preserving the original aspect ratio.
See the following example.
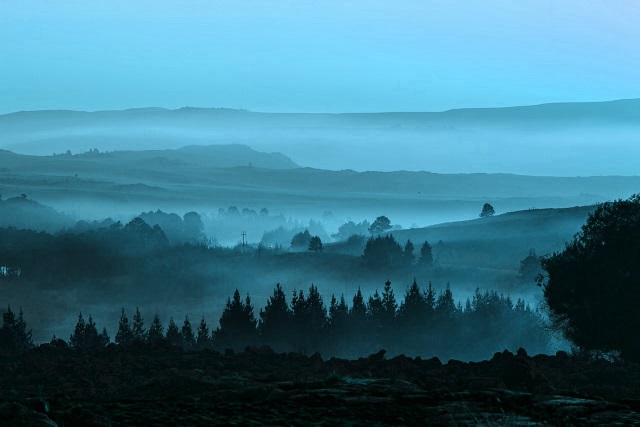
<div class="row"> <div class="col flex justify-content-center"> <img src="/webui/assets/images/landscape_blue.jpg" class="image" /> </div> </div>
You can also change the aspect ratio of an element (which may contain an image) using one of the media-container-* classes and a media-content class. The following example sets the aspect ratio to 21x9.
<div class="responsive-gutter-lg"> <div class="media-container-21x9 border-sm border-info"> <div class="media-content info-dark"></div> <label class="place-top-left pad-sm info text-bold">21x9</label> </div> </div>
Adding Color
To add a foreground color to any element use the color-* classes.
To add a background color to any element use the background-* classes.
Here are some examples with additional hover event changes.
The -hover- section of the class name can be replaced with -focus- to make colors apply when focusable elements such as form controls receive focus.
<div class="row gap-sm col-padding-sm text-bold text-center"> <div class="col border-sm border-default color-accent-3 background-accent-3-dark background-hover-light rounded-sm"> Lorem ipsum </div> <div class="col border-sm border-default color-light color-hover-warning background-dark-alternate rounded-sm"> Lorem ipsum </div> <div class="col border-sm border-default color-dark background-accent-1 color-hover-info-light rounded-sm"> Lorem ipsum </div> <div class="col border-sm border-default color-warning-dark background-warning-light background-hover-warning rounded-sm"> Lorem ipsum </div> <div class="col border-sm border-default color-hover-light color-hover-success rounded-sm"> Lorem ipsum </div> </div>
For more information on using theme colours see the Colours section.
Accessibility
Accessibility Focus Ring
WebUI provides the accessibility-* classes to increase the visibility of form controls. This behaviour relates to control focus events. You can specify a theme color for the accessibility focus ring.
Adding an accessibility-* class to a parent element will cause all focusable child controls to show a focus ring when tabbed to or gaining focus. The class cannot be applied to individual controls.
<div class="col-padding-lg accessibility-accent-4"> <div class="row"> <div class="col flex-items-center gap-horizontal-md"> <label>First name</label> <input name="firstName" type="text" class="input-sm width-md-lg rounded-sm" /> </div> </div> <div class="row"> <div class="col"> This demonstrates an <a href="#0" class="color-info text-underline"> Accessible Hyperlink</a>, improving visibility when focused. </div> </div> </div> <div class="col-padding-lg control-group-success accessibility-accent-4"> <div class="row"> <div class="col flex-items-center gap-horizontal-md"> <label>First name</label> <input name="firstName" type="text" class="input-sm width-md-lg rounded-sm" /> </div> </div> <div class="row"> <div class="col"> This demonstrates an <a href="#0" class="color-info text-underline"> Accessible Hyperlink</a>, improving visibility when focused. </div> </div> </div>
Accessibility Classes
List of accessibility classes available.
Class | Description |
---|---|
accessibility | Adds a focus ring to focusable elements when receiving focus, and in the default theme color. |
accessibility-light | Adds a focus ring to focusable elements when receiving focus, and in the light theme color. |
accessibility-dark | Adds a focus ring to focusable elements when receiving focus, and in the dark theme color. |
accessibility-accent-1 | Adds a focus ring to focusable elements when receiving focus, and in the accent-1 theme color. |
accessibility-accent-2 | Adds a focus ring to focusable elements when receiving focus, and in the accent-2 theme color. |
accessibility-accent-3 | Adds a focus ring to focusable elements when receiving focus, and in the accent-3 theme color. |
accessibility-accent-4 | Adds a focus ring to focusable elements when receiving focus, and in the accent-4 theme color. |
accessibility-accent-5 | Adds a focus ring to focusable elements when receiving focus, and in the accent-5 theme color. |
screen-reader | Causes an element to be hidden, but still available to assistive technologies. |
ARIA Attributes
WebUI controls make use of ARIA accessibility attributes where it would be difficult for a website author to add them. However, most of these attributes should be added to elements during development of a website. See MDN web docs - ARIA for more information.
Keyboard Navigation
All WebUI components such as menus, tabs, and modals can be activated using the default browser shortcuts. For example: Custom checkboxes and radio buttons can be tabbed to and selected / deselected using the spacebar. The tabs component allows navigation between tabs using the tab key and selected using the enter key. A lot depends on using appropriate controls, and to make the menu component accessible you need to use the activator-focus class on menu items.